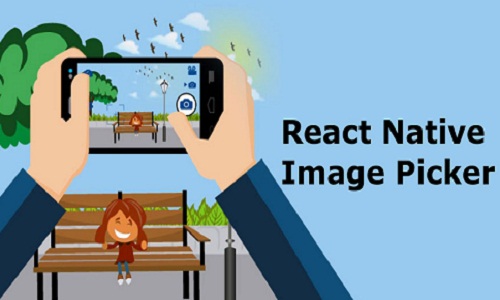
The React native app development company have built several cross-platform apps just using the React Native framework. In this blog, I will discuss how to use the React Native framework to make an image picker app. Moreover, you will get a shortcut to simplify your code by integrating the React Native third-party plugin support.
Let’s see what the prerequisite parameters that you need to meet are.
Prerequisites Parameter
- Firstly, you have to get all the necessary dependencies installed in your system. With this, I mean to say, you need to set up your development system with the required RN environment. If you are looking for related guidance,
- You have to learn about the utility of GET requests and API calling methods. For the live data of the weather, you have to fetch data from a specific web server.
Third-Party Plugin- React-Native-Image-Crop-Picker
As you are familiar with, React Native framework supports third-party native libraries. You don’t need to burden your app with additional components and functionalities. If the React Native framework does not support functionality such as Google Maps, you can easily install the third-party library and use the native components in your app. This not only allows users to compromise with other mobile apps but also lets developers extract code syntax and add it to their codebase. For this project, you do need to add a third-party React Native plugin: react-native-image-crop-picker in your root directory.
Note, that there are numerous third-party packages that you can install in your app directory, you have to be specific about the need and its utility for the project. Let me give you an example. I could have used react-native-image-picker in this project but I have used the react-native-image-crop-picker in my codebase. The former would not allow you to crop the chosen image in the specified frame; however, the latter will let you choose images, compress images, edit the same, and crop as per the fit of the frame.
You can find other packages stored as dependencies in the ./package.json folder. Check the GitHub repository of this project for the complete code.
As you have become familiar with the significance of the third-party React Native plugins for this app, let’s now start with the explanation of the code snippets.
Interpretation of Code Snippets
import { Button, StyleSheet, Text, View, Image } from ‘react-native’
import React,{useState,useEffect} from ‘react’
import ImagePicker from ‘react-native-image-crop-picker’;
Here, I imported all the required components for the current project. Button, Text, Image, View, and StyleSheet are imported from the ‘react-native’ package. You need to use the View component to hold different containers. Text is for getting text elements. The StyleSheet is to style the container, button, or other elements integrated into the app. Button component is used for getting Pressable buttons. Lastly, the Image is the most important component since we need to pick an image from the local folder.
navigator.geolocation = require(‘@react-native-community/geolocation’);
const App = () => {
const [img,setImg]=useState()
const Handle=()=>{
ImagePicker.openPicker({
width: 300,
height: 400,
cropping: true
}).then(image => {
setImg(image)
});
}
The geolocation navigator is taken from the package ‘@react-native-community/geolocation’. It will allow users to enable geolocation in the background of the app. With this line const App = () => {…}, I have created a constant variable App. Further, you need to define what elements or designs you want to integrate with. As you can see, the useState hook is used in the next line. If called, the hook will return two items. Here, it is the img and setImg. The former one is the state variable and the other one is the function that you use for updating the value of the defined state variable, which is the img. A constant variable Handle function is introduced. This is to open the ImagePicker. Further, the width and height of the Picker container is set as 300 pixels and 400 pixels respectively. I enabled the cropping as true. You need to use setImg() function to hold the chosen image from the local folder.
const cam=async()=>{
const img= await ImagePicker.openPicker({
multiple: true
})
console.log(img,’21’)
}
With the async()=>{…} syntax, I created a constant variable cam function. I used the console.log() function to print the image chosen from the local folder.
return (
<View>
{ img &&
<Image source={{uri:img.path}} style={styles.img}/>
}
<View style={styles.oneImage}>
<Button title=’take one Image’ onPress={()=>{Handle()}}/>
</View>
<View>
</View>
</View>
)
}
export default App
const styles = StyleSheet.create({
oneImage:{
marginVertical:20,
width:200,
height:35,
marginHorizontal:100
},img:{width:200,
height:200,
borderRadius:50,
marginVertical:10,
marginHorizontal:100}
})
- Here, the <View> component holds two child components: Button and Image. The Button has a title “take one Image” which is clickable. On pressing the button, the onPress() function is enabled and it will further call the Handle() I set the Image source as {uri:img.path}.
- You have to use the StyleSheet component to design both the img and Refer to the code snippets after the const styles = StyleSheet.create({
As we are done with the coding and building part. Let’s now see how you can start the emulator and run the app on your virtual device.
Steps to Run the Application
- Go to the command prompt. Run npm install. With this command, you will install all the needed dependencies.
- After it is done, run npx react-native run-android. You have to wait till the bundling is done. You will see a screen on the emulator as given in image 1.
Image 1
- On clicking the blue button, your local phone’s folder will open up. If you have no photos or images in your folder, you have to get a picture from the internet. For this, you have to sign up for your google account and download an image.
- Once you complete these steps, start the app from the first. Go to your local folder through the emulator and click one picture. You can see the selected image in a frame where you can crop further. Refer to image 2.
Image 2
- Press the right button. And you are successfully done with building and running the app.
Building an app and adding features to it is a no-brain game. However, the most important criterion that you need to achieve is to gain proper knowledge about the React Native framework and the utility of its different components.
Be the first to comment